If you find this helpful, please click the Google |


Web Site Template Design Pattern
HTML Design Pattern for a Web Site Template
A web site template can be applied to multiple web pages to avoid repeating the code for common elements on all web pages. It can also be cached on the client side by web browsers, to improve page load times. For example, you can have a style sheet with templates that create site logos and navigation, which can be updated to easily change the "look and feel" of the entire web site.
This site is an actual working example of a web site with a site-wide template. If you do "View Source" on this page, you will not see the code for the logo at the top, the navigation section on the left or various other common elements, which appear in the same location on all pages of the site, because they are loaded from the site template.
Chrome is a good browser for developing templates because its Developer Tools show the HTML code after the template has been applied to the page. For example, in the picture below you can see the unordered list and list items for the navigation links.
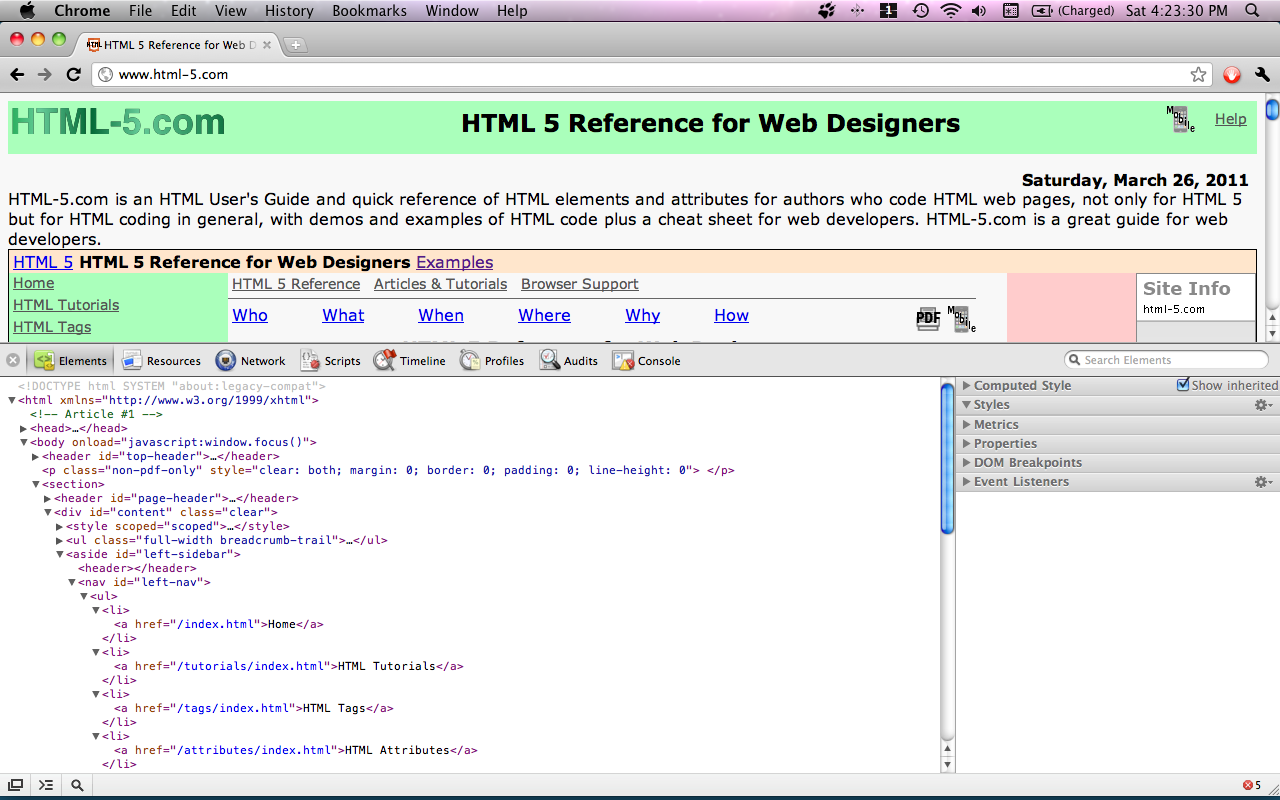
Web Site Template Design Pattern Code
HTML and XSL Code for a Web Site Template
In the HTML code for each page of the web site, include an <?xml-stylesheet?> instruction referencing the site's style sheet file.
<?xml version="1.0" encoding="UTF-8"?> <?xml-stylesheet type="text/xsl" href="<#/path/template-name.xsl#>"?> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title><#Page Title#></title> </head> <body> ... see other HTML Design Patterns ... </body> </html>
Create a single style sheet file at the location specified by the <?xml-stylesheet href?> attribute.
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:html="http://www.w3.org/1999/xhtml" xmlns="http://www.w3.org/1999/xhtml" exclude-result-prefixes="xsl html" > <!-- Copyright 2011 Accilent Corp. Permission to use granted. Provided AS IS, no warranties. --> <xsl:output method="xml" version="1.0" omit-xml-declaration="no" indent="yes" doctype-system="about:legacy-compat"/> <xsl:template match="/"> <!-- removes stylesheet processing option --> <xsl:copy> <xsl:apply-templates select="*"/> </xsl:copy> </xsl:template> <xsl:template match="/html:html/html:body"> <xsl:copy> <xsl:apply-templates select="@*"/> <header> <#HTML code for header#> </header> <xsl:apply-templates select="node()"/> <footer> <#HTML code for footer#> </footer> </xsl:copy> </xsl:template> <!-- requires <nav id="left-nav"></nav> where navigation is to appear --> <xsl:template match="html:nav[@id='left-nav']"> <xsl:copy> <xsl:apply-templates select="@*"/> <ul> <li><a href="/index.html">Home</a></li> <li><a href="/<#another-page.html#>"><#Link Text#></a></li> <li><a href="/<#subdirectory#>/"><#Link Text#></a></li> <#more navigation links#> </ul> <xsl:apply-templates select="node()"/> </xsl:copy> </xsl:template> <xsl:template match="@*|node()"> <xsl:copy> <xsl:apply-templates select="@*|node()"/> </xsl:copy> </xsl:template> </xsl:stylesheet>
The match="/html:html/html:body"
template shows an example of how to include content based on the location of a unique HTML element in the source document. In this case the template is adding some HTML header code and some footer code to the <body> element.
The match="html:nav[@id='left-nav']"
template shows an example of how to include content based on the location of an element with a specific id
attribute. In this case the template is adding a series of site-wide navigation links on the left hand side of each page.
Web Site Template Examples
Examples of web site templates in HTML
HTML document with a site template
<?xml version="1.0" encoding="UTF-8"?> <?xml-stylesheet type="text/xsl" href="/site-template.xsl"?> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Example Only</title> </head> <body> <p>Hello World!</p> </body> </html>
Web site template example
The template code is stored in a separate .xsl (extensible style sheet language) file. For the example code above, the file is located in the document root directory of the web server (/
) and is named site-template.xsl
.
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:html="http://www.w3.org/1999/xhtml" xmlns="http://www.w3.org/1999/xhtml" exclude-result-prefixes="xsl html" > <!-- Copyright 2011 Accilent Corp. Permission to use granted. Provided AS IS, no warranties. --> <xsl:output method="xml" version="1.0" omit-xml-declaration="no" indent="yes" doctype-system="about:legacy-compat"/> <xsl:template match="/"> <!-- removes stylesheet processing option --> <xsl:copy> <xsl:apply-templates select="*"/> </xsl:copy> </xsl:template> <xsl:template match="/html:html/html:body"> <xsl:if test="not(.//html:header[@id='top-header'])"> <xsl:call-template name="top-header"> <xsl:with-param name="style" select="'background-color: #aaffbb'"/> </xsl:call-template> </xsl:if> <xsl:copy> <xsl:apply-templates select="@*|node()"/> <footer id="page-footer"> <nav> <a href="/contact-us.html">My Contact Info</a> </nav> </footer> </xsl:copy> </xsl:template> <xsl:template match="html:header[@id='top-header']"> <xsl:copy> <xsl:apply-templates select="@*"/> <xsl:call-template name="top-header"> <xsl:with-param name="style" select="'float: left'"/> </xsl:call-template> <xsl:apply-templates select="node()"/> </xsl:copy> </xsl:template> <xsl:template name="top-header"> <xsl:param name="style"/> <div id="top-logo" style="{$style}"> <a href="/" onfocus="this.blur()"> <img src="/site-logo.png" width="222" height="66" alt="ExampleOnly.com"/> </a> </div> </xsl:template> <!-- requires <nav id="left-nav"></nav> where navigation is to appear --> <xsl:template match="html:nav[@id='left-nav']"> <xsl:copy> <xsl:apply-templates select="@*"/> <ul> <li><a href="/index.html">Home</a></li> <li><a href="/another-page.html">Another Page</a></li> <li><a href="/subdirectory/">More Info</a></li> </ul> <xsl:apply-templates select="node()"/> </xsl:copy> </xsl:template> <xsl:template match="@*|node()"> <xsl:copy> <xsl:apply-templates select="@*|node()"/> </xsl:copy> </xsl:template> </xsl:stylesheet>